Fixing a 10-year-old bug in Firefox’s native input validation and implementing custom controls for improved frontend development
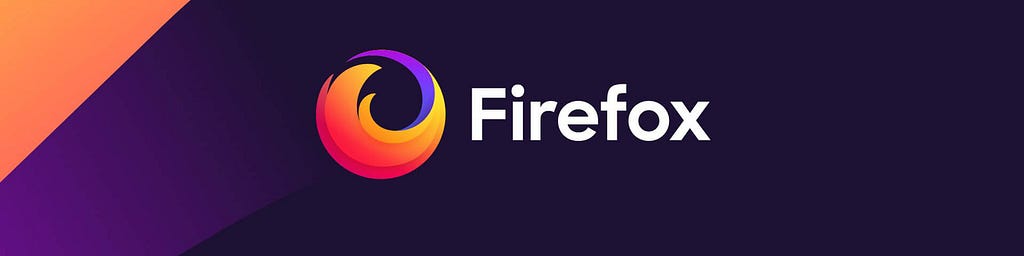
(Apr 17, 2021 — original article from https://lukasz.moskwa.dev/posts/a-bug-meant-to-stay)
A few years ago, I remember working as a web developer for an IT company, and my job was to redo their website following the directions of the design department. It was a mostly mechanical job since the specifications I was given were precise and accurate. The old page was rendered server-side via ASP.NET Core without any frameworks, and since the new page was to be built with Angular 5, there wasn’t much material to take from.
In the meantime, a lot of the functionality had also changed from the old site: for example, there were new entries and new controls to do among the registration and search parameters. This was also true for all the forms.
As we know, form validation is a fundamental part of frontend development. It allows standardizing the data sent to the backend, preventing the sending of information in the wrong format as much as possible. Moreover, if done well, it improves the experience of the user who uses the portal, notifying him of any errors in the compilation and how to correct them.
The Code
When making the registration form, I remember following all the design guidelines to comply with the mockup and preparing the submit function. Since there were no design specs on the wrong inputs and the controls to be made were straightforward, I naively thought of using HTML5’s native controls. Yes, these.
<!DOCTYPE html>
<html>
<body>
<h1>The input required attribute</h1>
<form action="/action_page.php">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<input type="submit">
</form>
</body>
</html>
I didn’t think anything was wrong, and after verifying in the browser that the behavior was what I expected, I went ahead with the development.
After a few days, I received an email from a tester reporting an error on that form. From the error description, his browser glitched when he entered the wrong data in one of the inputs. The error was very specific: the “bubble” reporting the invalid field did not point to the same field as the page scrolled. As trivial as it may seem, it’s still an inaccuracy and needs to be fixed.
Initially, I didn’t understand how it was possible that it didn’t work: I assumed that the CSS of the page was somehow fighting (and losing) against the native components of the browser. This thing was only happening on Firefox. I could have understood similar behavior on Safari, but seeing it on my favorite browser had shattered some certainties.
Once I concluded that it couldn’t be the CSS (none of my changes affected the final result on the scroll), I searched the Internet to see if anyone else had encountered the same problem. Maybe it was the version of Firefox. Maybe another factor I wasn’t considering.
The Hunt
Despite the somewhat imprecise search query (I didn’t know how to describe the problem accurately), I began to come across the first questions on Stack Overflow that addressed this issue:
- Firefox HTML5 invalid form message bubble position
- Webkit Validation Bubble behaves like it has fixed position on Chrome
My best guess was that it was a bug. August 2015. Four years prior, and no update on the application since then. It was time to go even deeper into the rabbit hole because I didn’t want to believe I had to change the implementation because of an unfixed bug in one of the browsers.
1426737 – HTML5 Input Validation Tooltip is Fixed Position and Doesn’t Scroll With Its Input
This GIF reproduced the issue perfectly:
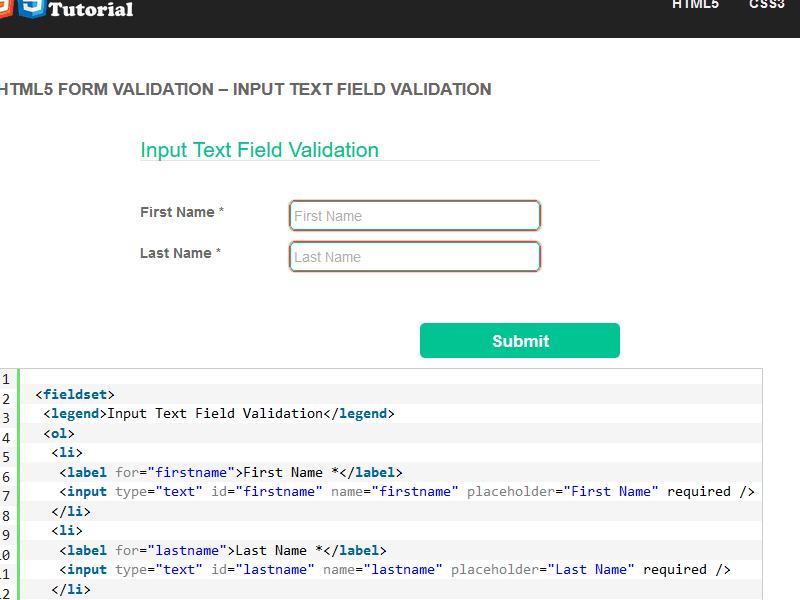
But it was this second link that shocked me the most.
643758 – [e10s] HTML5 validation popup doesn’t close when the form element scrolls
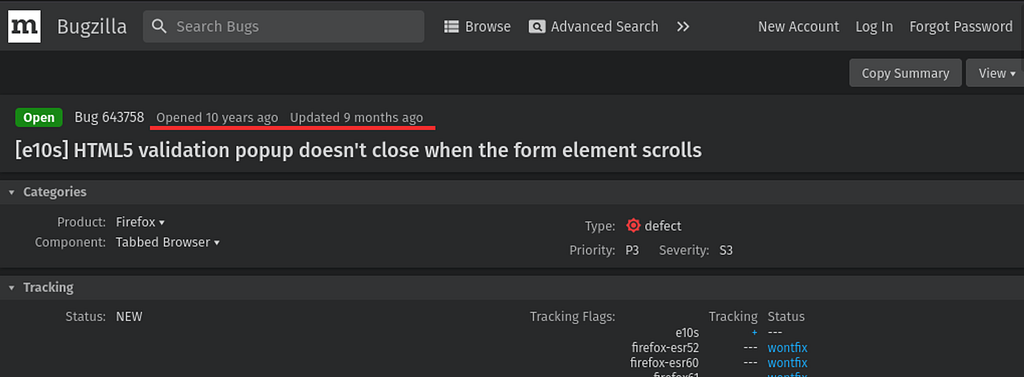
Ten… years? Okay, they definitely forgot about that one here. Although I wasn’t crazy about the idea, it was time to find a workaround, even if it was just for a browser.
The Fix
To prevent the user from entering wrong data or forgetting to enter data, it is necessary to implement the controls in another way. To do this, it is sufficient to use a JavaScript and CSS combo:
<!DOCTYPE html>
<html>
<style src="./style.css"></style>
<body>
<h1>The input required attribute</h1>
<form name="loginForm" action="/action_page.php" onsubmit="return validateForm()">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<input type="submit">
</form>
</body>
<script src="./validate.js"></script>
</html>
The CSS
.error {
border: 1px solid red;
color: red;
}
and the JavaScript code:
function validateForm(){
const formFields = document.forms["loginForm"]
for(const field in formFields){
if(formFields[field].value === ""){
formFields[field].classList.add("error")
} else {
formFields[field].classList.remove("error")
}
}
}
Overcoming Form Validation Issues in Firefox With HTML5 Workarounds was originally published in Better Programming on Medium, where people are continuing the conversation by highlighting and responding to this story.