A comprehensive Guide
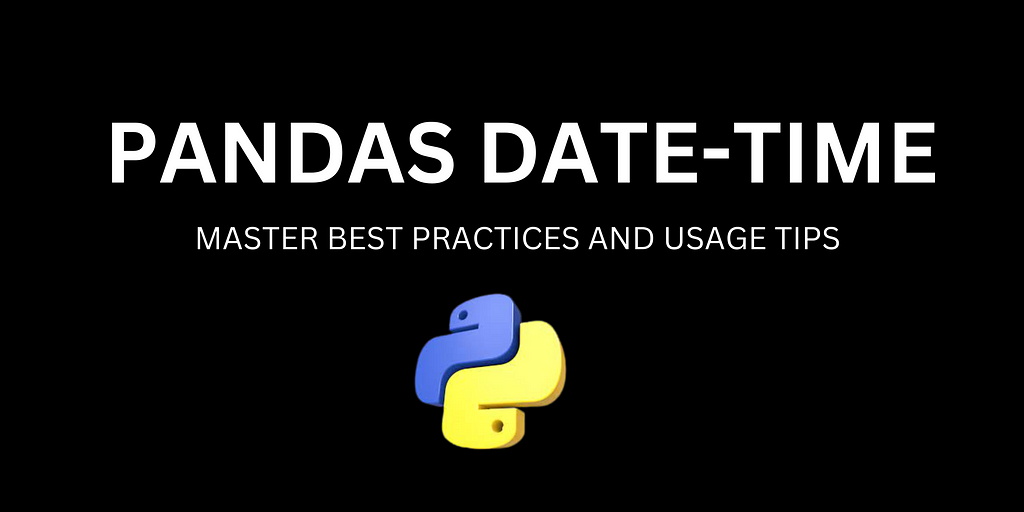
As a data analyst, working with time-related data is a common occurrence. Whether it’s evaluating stock prices, studying weather patterns, or analyzing user activity on a website, understanding and manipulating dates and times is essential. Luckily, Pandas, a widely-used Python library, offers a robust date-time functionality to help with this task. In this article, we will delve into the topic of Pandas’ date-time functionality, covering best practices and usage tips to work with time-related data effectively.
Prerequisites
To follow this article and work effectively with Pandas’ date-time functionality, you should be familiar with the following prerequisites:
- You should have a good understanding of Python programming, including variables, data structures, loops, and conditional statements.
- Familiarity with Pandas is essential, as you’ll be using Pandas to manipulate date and time data.
- Pandas build on top of NumPy, so having some knowledge of NumPy can be beneficial.
- Understand the basic concepts of date and time manipulation, such as dates, times, time zones, and formats.
- You should have Pandas and NumPy installed. You can use pip or conda for this purpose.
Outline
- What is Pandas’ date-time?
- Why Pandas’ date-time?
- How to use Pandas date-time
- Important tips and best practices
- Conclusion
What is Pandas’ date-time?
Pandas date-time refers to a functionality in the Pandas library that handles date and time data. Pandas is a widely used data manipulation and analysis library for Python, built on top of the NumPy library. It offers robust tools for working with structured data, including date and time data.
The date-time functionality of Pandas is remarkably useful for analyzing time series data, managing historical data, and more. It simplifies the process of working with date and time data in Python, making it effortless to manipulate, analyze, and visualize temporal data.
The main components of Pandas’ date-time functionality include:
- Date-time Index: Pandas allows you to create date-time indices, which are used to label and organize data in a time series. These indices can be used to efficiently select, filter, and aggregate data based on time.
- Date-time Data Types: Pandas provide data types like Timestamp for representing individual date and time values, and you can also work with Series and DataFrames containing date-time data.
- Date-time Parsing: Pandas can parse date and time data in various formats, making it easy to convert strings into date-time objects.
- Date-time Arithmetic: You can perform various arithmetic operations with date-time objects, such as addition, subtraction, and finding time intervals.
- Resampling: Pandas provides tools for resampling time series data at different frequencies (e.g., resampling daily data to monthly or annual data).
- Time Zone Handling: You can work with time zone information and perform conversions between different time zones.
- Date Ranges: Pandas allows you to generate date ranges, which can be useful for creating time-based sequences.
Why Pandas Date-time?
Pandas is an essential tool for data analysis in Python, and its date-time functionality is crucial for handling time series data. Some of the key reasons to use Pandas date-time are:
- Efficient Data Handling: Pandas date-time provides a flexible and efficient way to work with date and time data in tabular form, such as DataFrames.
- Data Alignment: It allows you to align data based on timestamps, making it easier to perform calculations and aggregations and merge data from different sources.
- Time Series Analysis: Pandas’ date-time is a foundation for time series analysis. You can easily perform operations like resampling, shifting, and rolling window calculations.
- Plotting and Visualization: Pandas integrates seamlessly with libraries like Matplotlib, making it easy to visualize time series data.
- Data Filtering: It allows you to filter and select data based on time ranges, which is useful for extracting specific time periods from your dataset.
How to use Pandas date-time
To work with Pandas date-time functionality, you’ll need to import the Pandas library. If you don’t have it installed, you can do so with the following command:
pip install pandas
Once Pandas is installed, you can import it and the date-time module into your Python script with the code below:
import pandas as pd
import datetime as dt
Now, you can review Pandas date-time module. To do this, you need to first create a date-time data with the code below:
today = dt.date(2023, 10 20)
Note that you are making use of Pandas date-time that you imported as dt to create a date-time variable. Also, note the format of the value that you are passing to the date-time object. In creating a date-time object with Pandas date-time, the year comes first followed by the month, and then the day, unless you state a different format.
Once you have date-time objects, you can access various components like year, month, day, hour, minute, second, and more using the object name followed by the component you want to access. For instance:
print(today.day)
print(today.month)
print(today.year)
This is a basic method you can use in creating a date-time object using Pandas date-time.
You can also create a time value for your date-time object by adding the code below:
today = dt.datetime(2023, 10, 20, 10, 33, 30)
If you try to print out the value of the today variable, with the code below,
print(today)
you will get a date and time object like the one below:
2023-10-20 10:33:30
You can also access each component of the time object by using the today variable followed by the time component you want to access:
print(today.hour)
print(today.minute)
print(today.second)
The value of the date-time object is expected to be numerical, but in some cases, you might have a variable that is a string, just like the one below
today = ("2023, 10, 20, 10:33:30")
if you witness such cases all you need is to convert the date-time object to a proper date-time value with pandas’ Timestap module like this:
DateTime = pd.Timestamp(today)
print(DateTime)
It is important to note that pandas’ Timestamp module works with a 24-hour time format, for instance, if you make use of the code below
today = pd.Timestamp("2023, 10, 20, 02:33:30 PM")
print(today)
Your time will be displayed as 14:33:30, because 02:33:30 PM represents 2:33 in the afternoon, which reads as 14:33, but if you use the code below:
today = pd.Timestamp("2023, 10, 20, 02:33:30 AM")
print(today)
Your time will be displayed as 02:33:30.
Sometimes you might have a list of data like the one below:
dates = ['2023, 10, 20', '2023, 10, 21', '2023, 10, 22']
In order to use the data above, you have to first convert it to a suitable date-time type with the help of DatetimeIndex module, like this:
pd.DatetimeIndex(dates)
This will give each data inside the list a date-time type. You can confirm this by checking dtype result that was returned and you will find out that you now have a date-time dtype instead of a list
Important tips and best practices
Using the Pandas date-time library for date and time data is quite common when working with time series data in Python. Here are some best practices and usage tips for working with Pandas date-time:
- Import Pandas and Convert Date/Time Columns: First, make sure to import the Pandas library and convert date and time columns to Pandas date-time objects.
- Set Date/Time Columns as Index: If your DataFrame represents time series data, consider setting the date/time column as the index. This can make time-based operations more efficient.
- Accessing Components: You can extract various components of a date-time object (e.g., year, month, day, hour, minute) using the name of the variable followed by the component you want to access.
- Calculations with date-time: You can perform various calculations with date-time objects, such as calculating time differences.
- Handling Missing Dates: If your time series data has gaps, you can use .asfreq() or .reindex() to fill in missing dates.
- Time Zone-Aware Arithmetic: When performing arithmetic with date-time objects in different time zones, be aware of time zone issues. Use the .dt accessor to perform operations in a time zone-aware manner.
- For large datasets, consider using the numpy.datetime64 data type for improved performance.
Conclusion
Pandas date-time is an invaluable tool for handling time-related data in Python. It offers a versatile and efficient way to work with time series data, enabling you to perform various operations, including resampling, filtering, shifting, and plotting. Whether you’re a data analyst, data scientist, or Python enthusiast, mastering Pandas date-time is a valuable skill that will enhance your ability to work with time-based data effectively.
In this article, I have explained some of the basic things Pandas date-time can do and some best practices and tips. As you explore time series data in your projects, you’ll discover many more capabilities and functions that make Pandas a powerful choice for working with dates and times. So, next time you encounter a dataset with time-related information, remember that Pandas date-time is here to help you make sense of it.
Master Pandas Date-time: Best Practices and Usage Tips was originally published in Better Programming on Medium, where people are continuing the conversation by highlighting and responding to this story.