Learn how to craft and display your first user-friendly tip in your SwiftUI app using Apple’s new TipKit framework.
TipKit is a framework to help you easily present helpful tips and educational cues to users, guiding them through the features of an app at the right moment. Designed for SwiftUI, UIKit, and AppKit, this article will specifically focus on SwiftUI.
In this post, I will refer to creating a tip for my app, Fussion, where you get Spotify’s Blend-like features for Apple Music. I want to create numerous tips to guide users to either add feature request, or learn how to use the app’s features.
Tip Protocol
Let’s delve into the protocol Tip, which conforms to both Identifiable and Sendable. This protocol acts as the foundation for creating, defining, and shaping tips.
@available(macOS 14.0, iOS 17.0, tvOS 17.0, watchOS 10.0, xrOS 1.0, *)
protocol Tip: Identifiable, Sendable { }
The Tip protocol has several instance properties that allow for different tip customisation. We start with the context of MyFirstTip, a simple tip, to explore how to set up the content of a tip.
struct MyFirstTip: Tip {
var title: Text {
Text("Testing a tip!")
}
var message: Text? {
Text("It is working!")
}
var image: Image? {
Image(systemName: "star")
}
}
Understanding the Properties
The foremost property is the title, a non-optional attribute and the main requirement for a tip that conveys the benefit of your tip. In the example of MyFirstTip, the title is “Testing a tip!”. Well, it is the first tip; we start with some testing, hah. This sets the stage and is often the first thing the user will read.
var title: Text { get }
Although optional, the next attribute, the message, works with the title to deepen user understanding. In MyFirstTip, this is presented as “It is working!” adding a layer of confirmation to the title that we are testing!
var message: Text? { get }
Then, we have the image, another optional property that provides visual context to the tip. It makes the tip more engaging while offering a quick, intuitive cue for users to grasp the essence of the tip. For MyFirstTip, this image of a star complements the title and message, making me happy about the testing process.
var image: Image? { get }
Each property — title, message, and image— serves its role in forming a valuable tip. The title catches your eye and grabs attention, the message makes things clear, and the picture adds a fun touch — these work together to create a helpful and easy-to-understand tip for the user.
Creating a Tip
A tip should be more than just informational — it should encapsulate the essence of the feature it’s guiding the user toward. I have created a WishKitTip tip to guide users on adding a “wish” or feature request within my app.
@available(macOS 14.0, iOS 17.0, tvOS 17.0, watchOS 10.0, *)
struct WishKitTip: Tip {
var title: Text {
Text("Add a Wish!")
.font(.tipTitle)
}
var message: Text? {
Text("Upvote or create your feature request for me to work on.")
.font(.tipMessage)
}
var image: Image? {
Image(systemName: "wand.and.rays.inverse")
}
}
Notice how I use SwiftUI’s built-in .font() modifier directly within the tip’s title and message properties. This demonstrates the advantage of TipKit’s full integration with SwiftUI—you can employ any SwiftUI modifiers for Text to make your tips customisable.
- title: Text: The title here is “Add a Wish!” with a font style set to .tipTitle. This aligns with the feature it discusses—adding a wish or feature request.
- message: Text?: The message serves as a secondary guide. It specifies what actions the user can perform—either upvote an existing wish or create a new one.
- image: Image?: The image uses the system symbol wand.and.rays.inverse, visually supporting the “wish” concept. An image can speak a thousand words, and here, it quietly but effectively tells the user that my coding magic to implement their favourite feature is just a click away. 🤪
Configuring TipKit
Before we show our first tip to users, we need to get TipKit ready. To do that, we use the Tips.configure() method. It configures an app’s tips.
@available(macOS 14.0, iOS 17.0, tvOS 17.0, watchOS 10.0, *)
@frozen public enum Tips {
public static func configure(_ configuration: [Tips.ConfigurationOption] = []) throws
}
Usually, you do this setup right when your app starts. For UIKit, this would be in the AppDelegate. For SwiftUI, you can do it directly in the @main App struct.
Here’s how I do it for my app, Fussion:
@main struct FussionApp: App {
init() {
do {
try await Tips.configure()
} catch {
print("Failed to configure TipKit: (error)")
}
}
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
With the initial setup done, we are ready to help us display useful tips to our users using SwiftUI.
Displaying the Tip
The TipKit team created TipView, making placing the views easier. We can add this TipView to any part of our app. I have a new tip in place — this time, I let the users know they can long-press to play a station.
@available(macOS 14.0, iOS 17.0, tvOS 17.0, watchOS 10.0, *)
struct PlayStationDirectlyTip: Tip {
var title: Text {
Text("Play the station!")
.font(.tipTitle)
}
var message: Text? {
Text("Long-press the cover art of the station to play it directly.")
.font(.tipMessage)
}
var image: Image? {
Image(systemName: "radio.fill")
}
}
Smart move, and it makes the navigation even simpler in Fussion:
ScrollView {
LazyVGrid(columns: columns) {
NavigationLink(destination: ///)
})
.buttonStyle(.plain)
}
.padding(.horizontal)
if #available(macOS 14.0, iOS 17.0, tvOS 17.0, watchOS 10.0, *) {
TipView(PlayStationDirectlyTip(), arrowEdge: .top)
.padding(.horizontal)
}
}
And there you have it! This way, the users are guided and enriched with features they might have missed.
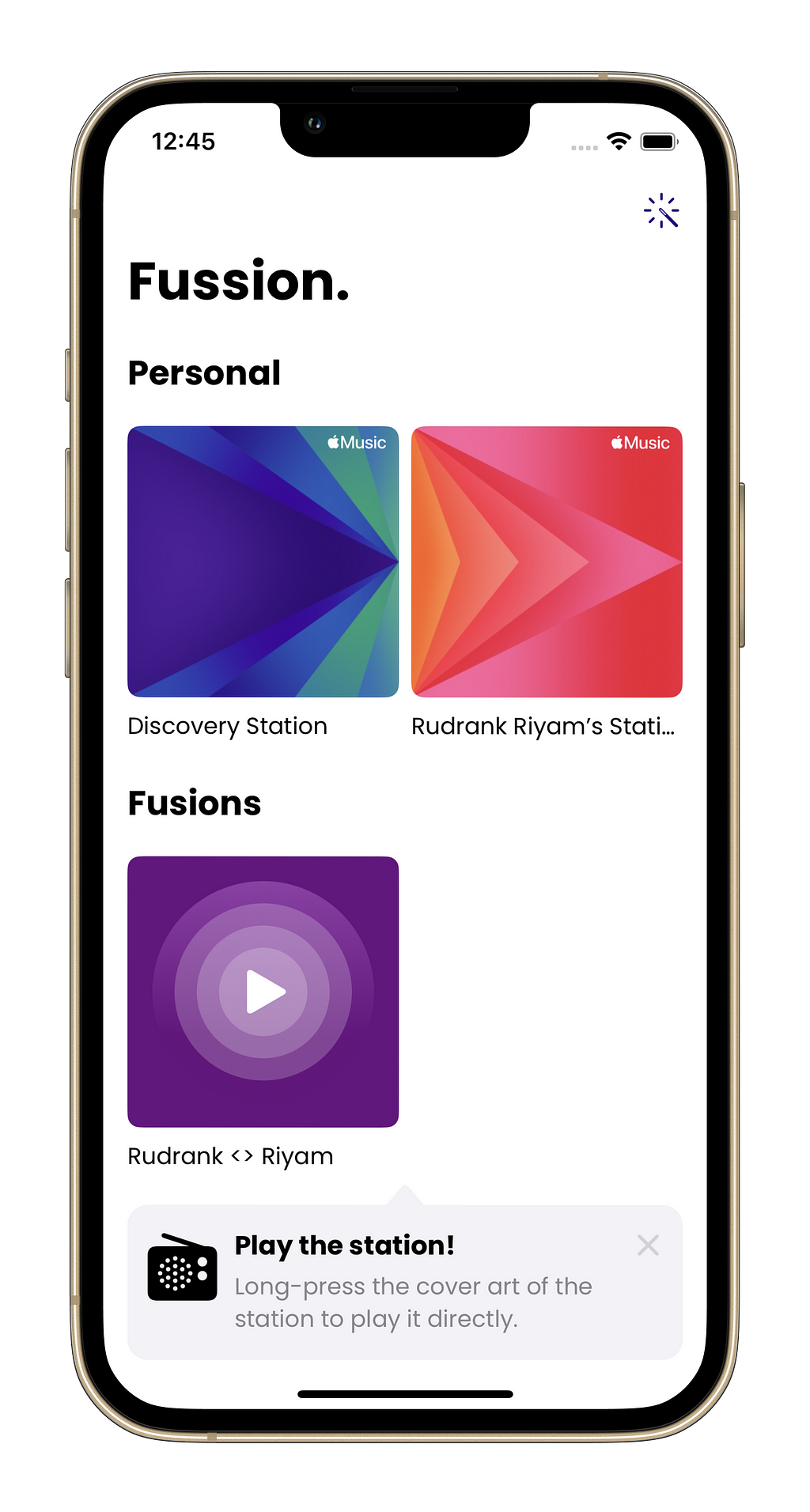
Conclusion
TipKit provides a straightforward yet powerful way to offer timely, context-sensitive tips in your apps. It supports all of Apple’s platforms and integrates cleanly with SwiftUI, UIKit, and AppKit. Properly setting the framework significantly improves your app’s user onboarding and feature discovery.
So, implement TipKit in your app and help users understand new features seamlessly. Happy coding!
Exploring TipKit: Creating Your First Tip was originally published in Better Programming on Medium, where people are continuing the conversation by highlighting and responding to this story.